Before you start
Next, let’s look at other ways to import Python code. Using and importlib to run Python code. Importmodule of importlib allows you to import and execute other Python scripts. The way it works is pretty simple. For our Python script code1.py, all we have to do is: import importlib import.importmodule(‘code1’). Jul 22, 2020 Run tests. Python tests.py Lint the project with; flake8 coderunner -max-line-length = 88-ignore = F401 black -check -diff coderunner 📝 Changelog. See the CHANGELOG.md file for details.:fire: Powered By. Judge0 API - Free, robust and scalable open-source online code execution system. 👥 Bhupesh Varshney. Twitter: @bhupeshimself.
Ensure that the following prerequisites are met:
You are working with PyCharm Community or Professional
You have installed Python itself. If you're using macOS or Linux, your computer already has Python installed. You can get Python from python.org.
To get started with PyCharm, let’s write a Python script.
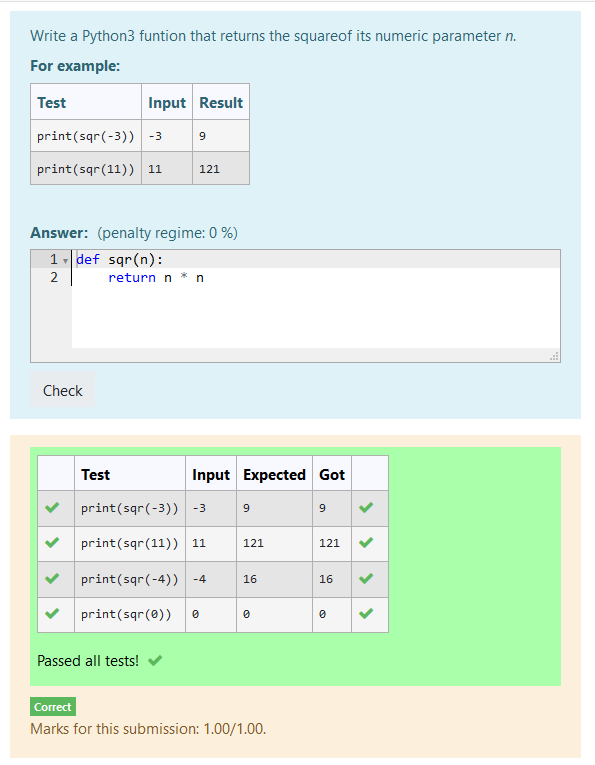
Create a Python project
If you’re on the Welcome screen, click New Project. If you’ve already got any project open, choose File | New Project from the main menu.
Although you can create projects of various types in PyCharm, in this tutorial let's create a simple Pure Python project. This template will create an empty project.
Choose the project location. Click button next to the Location field and specify the directory for your project.
Also, deselect the Create a main.py welcome script checkbox because you will create a new Python file for this tutorial.
Python best practice is to create a virtualenv for each project. In most cases, PyCharm create a new virtual environment automatically and you don't need to configure anything. Still, you can preview and modify the venv options. Expand the Python Interpreter: New Virtualenv Environment node and select a tool used to create a new virtual environment. Let's choose Virtualenv tool, and specify the location of the environment and the base Python interpreter used for the new virtual environment.
When configuring the base interpreter, you need to specify the path to the Python executable. If PyCharm detects no Python on your machine, it provides two options: to download the latest Python versions from python.org or to specify a path to the Python executable (in case of non-standard installation).
Refer to Configure a Python interpreter for more details.
Now click the Create button at the bottom of the New Project dialog.
If you’ve already got a project open, after clicking Create PyCharm will ask you whether to open a new project in the current window or in a new one. Choose Open in current window- this will close the current project, but you'll be able to reopen it later. See the page Open, reopen, and close projects for details.
Create a Python file
In the Project tool window, select the project root (typically, it is the root node in the project tree), right-click it, and select File | New ...
Select the option Python File from the context menu, and then type the new filename.
PyCharm creates a new Python file and opens it for editing.
Edit Python code
Let's start editing the Python file you've just created.
Start with declaring a class. Immediately as you start typing, PyCharm suggests how to complete your line:
Choose the keyword
class
and type the class name,Car
.PyCharm informs you about the missing colon, then expected indentation:
Note that PyCharm analyses your code on-the-fly, the results are immediately shown in the inspection indicator in the upper-right corner of the editor. This inspection indication works like a traffic light: when it is green, everything is OK, and you can go on with your code; a yellow light means some minor problems that however will not affect compilation; but when the light is red, it means that you have some serious errors. Click it to preview the details in the Problems tool window.
Let's continue creating the function
__init__
: when you just type the opening brace, PyCharm creates the entire code construct (mandatory parameterself
, closing brace and colon), and provides proper indentation.If you notice any inspection warnings as you're editing your code, click the bulb symbol to preview the list of possible fixes and recommended actions:
Let's copy and paste the entire code sample. Click the copy button in the upper-right corner of the code block here in the help page, then paste it into the PyCharm editor replacing the content of the Car.py file:
This application is intended for Python 3
class Car: def __init__(self, speed=0): self.speed = speed self.odometer = 0 self.time = 0 def say_state(self): print('I'm going {} kph!'.format(self.speed)) def accelerate(self): self.speed += 5 def brake(self): if self.speed < 5: self.speed = 0 else: self.speed -= 5 def step(self): self.odometer += self.speed self.time += 1 def average_speed(self): if self.time != 0: return self.odometer / self.time else: pass if __name__ '__main__': my_car = Car() print('I'm a car!') while True: action = input('What should I do? [A]ccelerate, [B]rake, ' 'show [O]dometer, or show average [S]peed?').upper() if action not in 'ABOS' or len(action) != 1: print('I don't know how to do that') continue if action 'A': my_car.accelerate() elif action 'B': my_car.brake() elif action 'O': print('The car has driven {} kilometers'.format(my_car.odometer)) elif action 'S': print('The car's average speed was {} kph'.format(my_car.average_speed())) my_car.step() my_car.say_state()
At this point, you're ready to run your first Python application in PyCharm.
Run your application
Use either of the following ways to run your code:
Right-click the editor and select Run 'Car' from the context menu .
Press Ctrl+Shift+F10.
Since this Python script contains a main function, you can click an icon in the gutter. If you hover your mouse pointer over it, the available commands show up:
If you click this icon, you'll see the popup menu of the available commands. Choose Run 'Car':
PyCharm executes your code in the Run tool window.
Here you can enter the expected values and preview the script output.

Note that PyCharm has created a temporary run/debug configuration for the Car file.
The run/debug configuration defines the way PyCharm executes your code. You can save it to make it a permanent configuration or modify its parameters. See Run/debug configurations for more details about running Python code.
Summary
Congratulations on completing your first script in PyCharm! Let's repeat what you've done with the help of PyCharm: Mad father mac.
Created a project.
Created a file in the project.
Created the source code.
Ran this source code.
In the next step, learn how to debug your programs in PyCharm.

In this tutorial, learn how to execute Python program or code on Windows. Execute Python program on Command prompt or use Python IDLE GUI mode to run Python code.
Create your file in .py extension and execute using the step-step process given here. The steps are given here with pictures to learn in the easiest way.
How to Execute Python Program Using Command Prompt
If you want to create a Python file in .py extension and run. You can use the Windows command prompt to execute the Python code.
Here is the simple code of Python given in the Python file demo.py. It contains only single line code of Python which prints the text “Hello World!” on execution.
So, how you can execute the Python program using the command prompt. To see this, you have to first open the command prompt using the ‘window+r’ keyboard shortcut. Now, type the word ‘cmd’ to open the command prompt.
This opens the command prompt with the screen as given below. Change the directory location to the location where you have just saved your Python .py extension file.
You can use the cmd command ‘cd’ to change the directory location. Use ‘cd.’ to come out of directory and “cd” to come inside of the directory. Get the file location where you saved your Python file.
To execute the Python file, you have to use the keyword ‘Python’ followed by the file name with extension. See the example given in the screen above with the output of the file.
You can also execute the code directly in the interactive mode with the next section.
Interactive Mode to Execute Python Program
To execute the code directly in the interactive mode. You have to open the interactive mode. Press the window button and type the text “Python”. Click the “Python 3.7(32 bit) Desktop app” as given below to open the interactive mode of Python.
Code-runner.executormap Python
You can type the Python code directly in the Python interactive mode. Here, in the image below contains the print program of Python.
Press the enter button to execute the print code of Python. The output gives the text ‘”Hello World!” after you press the enter button.
Type any code of Python you want to execute and run directly on interactive mode.

However, you can also execute the Python program using the Python GUI. You have to use the below section and follow the examples with pictures.
Using IDLE(Python GUI) to Execute Python Program
Another useful method of executing the Python code. Use the Python IDLE GUI Shell to execute the Python program on Windows system.
Open the Python IDLE shell by pressing the window button of the keyboard. Type “Python” and click the “IDLE(Python 3.7 32 bit)” to open the Python shell.
Create a Python file with .py extension and open it with the Python shell. The file looks like the image given below.
It contains the simple Python code which prints the text “Hello World!”. In order to execute the Python code, you have to open the ‘run’ menu and press the ‘Run Module’ option.
or
You can also use the keyboard shortcut ‘F5’ to run the Python code file.
A new shell window will open which contains the output of the Python code. Create your own file and execute the Python code using this simple method using Python IDLE.
Code Runner Python 3
A Python IDLE mode can also execute the direct code.
To execute the direct code, you have to write the Python code directly on the Python IDLE shell. Here, a print function of Python prints the text “Hello World” on execution.
Code Runner For Python Download
Hope, you like this tutorial of how to run Python program on windows.